
August 7, 2018 12:00 by
Peter
In this article we will be seeing how to create a Custom Silverlight UserControl and Binding the Property. We will use a dependency Property in this example.
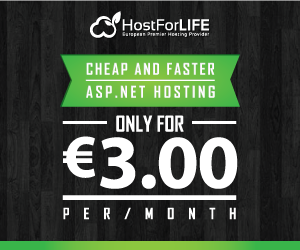
Created a Project as SamplePro.
Added an a new User Control as MyNewControl.
Dependency Property:
Now let's add a dependency property. To know more about DP, check out this site:
http://msdn.microsoft.com/en-us/library/cc221408(v=vs.95).aspx
My dependency property code looks as follows:
// Dependency Property - Begin
public const string DisplayTextPropertyName = "DisplayText";
public string DisplayText
{
get
{
return (string)GetValue(DisplayTextProperty);
}
set
{
SetValue(DisplayTextProperty, value);
}
}
public static readonly DependencyProperty DisplayTextProperty = DependencyProperty.Register(
DisplayTextPropertyName,typeof(string), typeof(MyNewControl),new PropertyMetadata(String.Empty));
// Dependency Property - End
The UserControl MyNewControl looks as below:
public partial class MyNewControl : UserControl
{
// Dependency Property - Begin
public const string DisplayTextPropertyName = "DisplayText";
public string DisplayText
{
get
{
return (string)GetValue(DisplayTextProperty);
}
set
{
SetValue(DisplayTextProperty, value);
}
}
public static readonly DependencyProperty DisplayTextProperty = DependencyProperty.Register(
DisplayTextPropertyName,typeof(string), typeof(MyNewControl),new PropertyMetadata(String.Empty));
// Dependency Property - End
public MyNewControl()
{
InitializeComponent();
btn.SetBinding(Button.ContentProperty, new Binding() { Source = this, Path = new PropertyPath("DisplayText"), Mode = BindingMode.TwoWay });
}
}
MyNewControl is ready for use as shown below:
<UserControl x:Class="SamplePro.MainPage"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:SamplePro"
mc:Ignorable="d"
d:DesignHeight="300" d:DesignWidth="400">
<Grid x:Name="LayoutRoot" Background="White">
<local:MyNewControl Width="300" Height="300" Foreground="Black" x:Name="newcontrol" DisplayText="Hit Me Now !!!"> </local:MyNewControl>
</Grid>
</UserControl>
Let's give it a run:
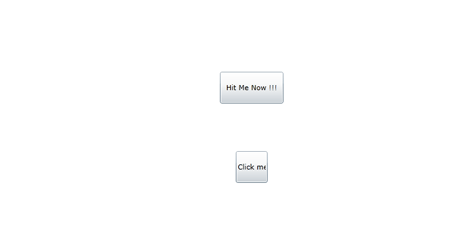